Cheating in a drawing game with Python
Gartic Phone is an online game that combines Pictionary with the Telephone game. In the game, players take turns drawing a word or sentence and guessing what others have drawn. Players use their mouse or a tablet-pen to draw on the screen.
I had some fun making a python program that simulates mouse events, just for the sake of impressing my friends with perfectly drawn geometrical shapes, which is quite a challege if done by hand.
Converting SVG paths to lists of points permalink
I used the svgpathtools
library to read an SVG image file and convert its paths into lists of points. Straight lines between these points would be an approximation of the path curves, and the point density can be adjusted in the SAMPLES_PER_PX
variable.
def svg_to_lines(img):
"""Read an svg file and calculate a list of points (x, y) for each path found.
Return a list of lists.
"""
SAMPLES_PER_PX = 0.3
paths, attributes, svg_attr = svgpathtools.svg2paths2(img)
lines = []
for path in paths:
amount = int(path.length() * SAMPLES_PER_PX)
points = []
for i in range(amount):
try:
point = path.point(path.ilength(path.length() * i / (amount-1)))
points.append((int(point.real), int(point.imag)))
except:
pass
lines.append(points)
return lines
Emulating Mouse Events Using PyAutoGUI permalink
Having our SVG read and converted to a list of points, we can start emulating mouse events. To do this, I used the pyautogui
library, which provides a cross-platform interface for simulating mouse and keyboard events.
The main drawing logic of the program is a loop that iterates through each line of points generated by the svg_to_lines
function. It moves the mouse to the first point of the line, presses the mouse down, moves the mouse to each subsequent point, and releases the mouse. The time.sleep
function is used to introduce a delay between each line to allow the browser to render the lines.
for n, line in enumerate(lines):
pyautogui.moveTo(*line.pop(0))
pyautogui.mouseDown()
for i, point in enumerate(line):
if i % 2 != 0:
continue
pyautogui.moveTo(*point)
pyautogui.mouseUp()
time.sleep(0.3)
Wrapping up permalink
In the video, you can see how the program transferred an image created in Inkscape to Gartic Phone. Did this serve any practical purpose? Perhaps not, but it certainly provided me with some entertainment as I caught my friends off guard with my precise shapes. Moreover, the process of developing the program was a stimulating challenge that I thoroughly enjoyed!
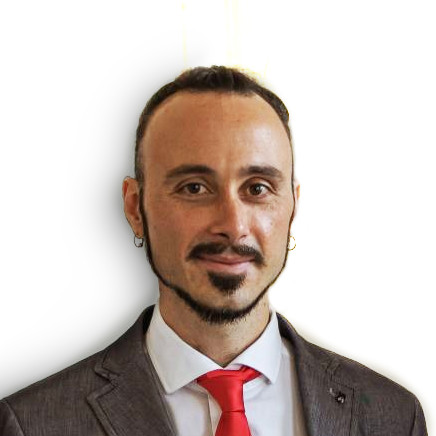